Java Random: Generating Numbers with java.util.Random
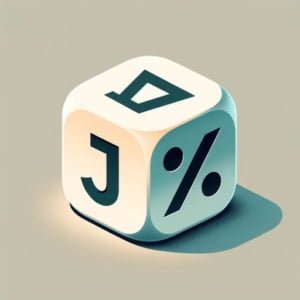
Ever been puzzled by generating random numbers in Java? You’re not alone. Many developers find themselves intrigued by the concept of randomness in programming. Think of Java’s Random class as a digital dice roller – capable of producing a stream of pseudorandom numbers.
This guide will walk you through the process of generating random numbers in Java, from the basics to more advanced techniques. We’ll cover everything from using the java.util.Random class, generating numbers within a specific range, to discussing alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering Java Random Number Generation!
TL;DR: How Do I Generate Numbers with java.util.Random?
To generate random numbers with the Random class in Java, it must be imported with,
import java.util.Random;
and instantiated with,Random rand = new Random();
Once created, you can then create your desired code. Here’s a simple example:
import java.util.Random;
Random rand = new Random();
int diceRoll = rand.nextInt(6) + 1;
System.out.println(diceRoll);
# Output:
# [Random integer between 1 and 6]
In this example, we import the java.util.Random
class and create a new instance of Random
called rand
. We then use the nextInt()
method to generate a random integer. The System.out.println(number);
line prints this random number to the console.
This is just the basic usage of the Random class in Java. There’s much more to learn about generating random numbers, including generating numbers within a specific range and using alternative methods. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with Java Random Class
- Generating Random Numbers Within a Specific Range
- Exploring Alternative Methods for Java Random Number Generation
- Troubleshooting Common Issues in Java Random Number Generation
- Understanding Random Number Generation
- Expanding the Horizon: Applications and Further Learning
- Wrapping Up: java.util.Random Class
Getting Started with Java Random Class
The Random class in Java is part of the java.util package. It allows us to generate random numbers, which can be very useful in a variety of scenarios like testing, gaming, or in any situation where you need to simulate a random outcome.
Here’s how you can use it to generate a basic random number:
import java.util.Random;
Random rand = new Random();
int number = rand.nextInt();
System.out.println(number);
# Output:
# [Random integer]
In this code snippet, we first import the java.util.Random
class. Then we create a new instance of Random
called rand
. The nextInt()
method is then used to generate a random integer, which is stored in the number
variable. Finally, we print the random number to the console using System.out.println(number);
.
The nextInt()
method generates a random integer in Java. However, it’s important to note that this method can return any integer, positive or negative. If you want to generate a positive random number, you can use the nextInt(int bound)
method, which we will discuss in the next section.
Remember, the numbers generated by the Random class are actually pseudorandom numbers. They are not truly random because they are determined by a mathematical algorithm. However, for most purposes, they are random enough.
Generating Random Numbers Within a Specific Range
While the basic use of Java’s Random class is quite straightforward, there might be instances where you want to generate a random number within a specific range. For instance, you might want to simulate a roll of a six-sided die, which would require a random number between 1 and 6.
Java’s Random class provides a method for this very purpose: nextInt(int bound)
. This method takes an integer as a parameter and returns a random integer between 0 (inclusive) and the bound (exclusive).
Here’s how you can use it:
import java.util.Random;
Random rand = new Random();
int number = rand.nextInt(6) + 1;
System.out.println(number);
# Output:
# [Random integer between 1 and 6]
In this example, rand.nextInt(6) + 1
generates a random integer from 1 to 6, simulating a dice roll. The ‘+1’ offset is added because nextInt(6)
generates a number in the range 0-5, so adding 1 makes it 1-6 to correctly simulate the dice roll.
This method is particularly useful when you need to generate a random number within a certain range, such as simulating random events in a game or selecting a random element from an array.
Exploring Alternative Methods for Java Random Number Generation
While the Random class is a robust tool for generating random numbers in Java, it’s not the only game in town. Other methods such as the Math.random()
function and third-party libraries also offer unique advantages.
Using Math.random()
The Math.random()
function is a simple alternative to the Random class. This method returns a pseudorandom double greater than or equal to 0.0 and less than 1.0.
Here’s how you can use Math.random()
to generate a random number between 1 and 6:
int number = (int)(Math.random() * 6) + 1;
System.out.println(number);
# Output:
# [Random integer between 1 and 6]
In this example, Math.random() * 6
generates a random double between 0.0 (inclusive) and 6.0 (exclusive). Casting the result to an integer ((int)
) truncates the decimal part, resulting in an integer between 0 and 5. Adding 1 shifts the range to 1-6.
Leveraging Third-Party Libraries
Third-party libraries like Apache Commons Lang provide additional utilities for generating random numbers. For example, the RandomUtils
class offers methods to generate random integers, longs, doubles, and floats.
Here’s an example using RandomUtils
to generate a random integer between 1 and 6:
import org.apache.commons.lang3.RandomUtils;
int number = RandomUtils.nextInt(1, 7);
System.out.println(number);
# Output:
# [Random integer between 1 and 6]
In this example, RandomUtils.nextInt(1, 7)
generates a random integer between 1 (inclusive) and 7 (exclusive), effectively simulating a die roll.
Each of these methods has its own advantages and trade-offs. The Random class provides a wide range of methods for different types of random values. Math.random()
is a simple, no-fuss way to get a random double, but requires additional steps to generate integers or other types of random values. Third-party libraries like Apache Commons Lang can provide additional utilities and flexibility, but require adding an external dependency to your project.
Troubleshooting Common Issues in Java Random Number Generation
While Java’s Random class and other methods offer powerful tools for generating random numbers, they’re not without their quirks. Let’s discuss some common issues you might encounter and how to address them.
Dealing with Negative Numbers
The nextInt()
method of the Random class can generate any integer, positive or negative. If you need to generate only positive numbers, you can use the Math.abs()
method to get the absolute value of the generated number.
import java.util.Random;
Random rand = new Random();
int number = Math.abs(rand.nextInt());
System.out.println(number);
# Output:
# [Positive random integer]
In this example, Math.abs(rand.nextInt())
generates a random integer and converts it to a positive number if it’s negative.
Handling Large Numbers
If you need to generate a very large random number, you might run into the limits of the integer data type in Java. In this case, you can use the nextLong()
method of the Random class to generate a random long, which has a much larger range than an integer.
import java.util.Random;
Random rand = new Random();
long number = rand.nextLong();
System.out.println(number);
# Output:
# [Random long]
In this example, rand.nextLong()
generates a random long, which can be any value between -9,223,372,036,854,775,808 and 9,223,372,036,854,775,807, inclusive.
These are just a few examples of the issues you might encounter when generating random numbers in Java. With a clear understanding of the tools at your disposal and their potential pitfalls, you’ll be well-equipped to handle any random number generation task.
Understanding Random Number Generation
Random number generation is a crucial concept in programming. It’s used in a variety of applications, ranging from games and simulations to cryptography and statistical sampling. But what does it mean to generate a random number in a deterministic system like a computer?
Pseudorandom Numbers: The Heart of Java Random
In reality, what we refer to as random numbers in programming are actually pseudorandom numbers. These numbers are generated by an algorithm, so they’re not truly random in the strictest sense. However, they’re unpredictable enough for most practical purposes.
In Java, the java.util.Random
class uses a linear congruential generator as its pseudorandom number generation algorithm. When you create a new instance of the Random class, you can provide a seed value. This seed is the starting point for the sequence of pseudorandom numbers that the Random instance will generate. If you don’t provide a seed, the current time in milliseconds will be used.
import java.util.Random;
Random rand = new Random(123);
int number = rand.nextInt();
System.out.println(number);
# Output:
# 1534672271
In this example, we’re creating a new Random instance with a seed of 123. The nextInt()
method will always return the same first number (1534672271) for this seed. This predictability can be useful for debugging, but if you want different sequences each time your program runs, you should use the no-argument constructor to seed the generator with the current time.
The Significance of Randomness in Programming
Randomness has a multitude of uses in programming. In games and simulations, it can be used to create diverse and unpredictable scenarios. In cryptography, it’s essential for creating secure keys. In statistical sampling, it’s used to select a representative sample from a larger population. Understanding how to generate and use random numbers is an important skill for any programmer.
In the following sections, we’ll delve deeper into the practical applications of random number generation in Java, exploring more advanced techniques and considerations.
Expanding the Horizon: Applications and Further Learning
Random number generation in Java isn’t just a theoretical concept. It has practical applications that extend into larger projects, game development, simulations, and more. Let’s explore these applications and provide resources for further learning.
Randomness in Game Development
In game development, random number generation can be used to create diverse and unpredictable scenarios, enhancing the gaming experience. For instance, it can determine the spawn location of an enemy, the loot a player might find, or even the outcome of a player’s action.
Simulations and Randomness
Simulations often rely on random number generation to create realistic scenarios. For instance, a weather simulation might use random numbers to generate different weather patterns. A traffic simulation could use randomness to determine the arrival times of cars.
Further Resources for Mastering java.util.Random Class
Here are some additional resources to deepen your understanding of random number generation in Java and its applications:
- IOFlood’s article on Java Classes provides info on Java development with classes coverage.
Java Timer Essentials – Master Java timer mechanisms for scheduling tasks and executing them at specified times.
Java UUID Basics – Learn how to generate unique identifiers in Java using UUIDs for various applications.
Oracle’s Official Java Documentation is a great resource for the details of any Java class.
Baeldung’s Guide to java.util.Random provides a comprehensive overview of the Random class and its methods.
GeeksforGeeks Java Random Class Article offers detailed explanations and code examples for the Random class.
Remember, mastering any concept in programming requires not only understanding the theory but also applying it in practice. Don’t be afraid to experiment with different methods and scenarios to fully grasp the power and flexibility of Java’s Random class.
Wrapping Up: java.util.Random Class
In this comprehensive guide, we’ve delved into the process of generating random numbers in Java, exploring the versatility of the Random class, and discussing alternative methods for various scenarios.
We began with the basics, learning how to generate simple random numbers using the Random class. We then broadened our perspective to include generating random numbers within a specific range, which added another layer of complexity to our understanding. We also examined alternative approaches to random number generation, such as using the Math.random() function and leveraging third-party libraries.
Along the way, we addressed common challenges that you might encounter when generating random numbers in Java, such as dealing with negative numbers and handling large numbers. For each issue, we provided solutions and workarounds to ensure a smooth coding experience.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Random Class | Versatile, wide range of methods | Can generate negative numbers |
Math.random() | Simple, no external dependencies | Generates doubles, not integers |
Third-Party Libraries | Additional utilities, flexibility | Requires external dependencies |
Whether you’re just starting out with Java or you’re looking to level up your skills, we hope this guide has deepened your understanding of random number generation in Java and its practical applications.
With its balance of versatility and power, Java’s Random class is a valuable tool for any programmer. Happy coding!